Will Signals replace RxJs?
A Practical Guide to Understanding the Differences and Choosing the Right Tool for the Job
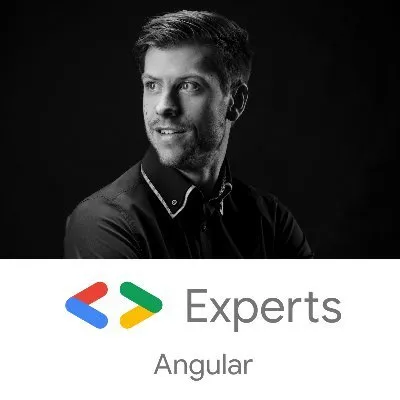
Kevin Kreuzer
@kreuzercode
Mar 13, 2025
4 min read

When Angular Signals were first introduced, many developers wondered: Will this make RxJS obsolete? The short answer is no — RxJS still has its place, especially for complex event streams and reactive workflows. However, for many common use cases, Signals provide a simpler alternative, making RxJS optional and, in many cases, unnecessary.
Interestingly, the Angular team has hinted that Observables may become optional in the future. That said, for RxJS to truly become optional, Angular needs new APIs that don’t rely on Observables. Currently, many built-in Angular APIs, such as HTTP requests and router events, return Observables by default. Until these APIs offer alternative ways to work with Signals, RxJS will continue to be a key part of Angular development.
We’re already seeing the emergence of new APIs that reduce the need for RxJS. One example is the new Resource API, which provides a way to fetch remote data without relying on Observables, making it more aligned with the Signal-based approach.
While Signals cover a lot of ground, there are still scenarios where RxJS excels and remains the better choice. Let’s take a closer look at those cases.
Where RxJS Still Shines
Signals have introduced a fresh way to handle reactive state in Angular, providing a more predictable and simplified reactivity model. However, when it comes to complex event handling, RxJS remains the go-to tool.
While Signals are great for managing state they are not designed for event handling. RxJS on the other hand is specifically designed for event-driven programming and excels in handling asynchronous streams of data.
In simple event handling scenarios, there might be no need for RxJS at all. However, as complexity increases — especially when dealing with user input events, API calls, and advanced data streams — RxJS and its powerful set of operators become indispensable.
Let’s consider a real-world example. Imagine a simple input field where users type a username, triggering an API request to fetch and display matching results. This use case may sound straightforward, but it has hidden complexities:
Requests should only fire if the input has more than a minimum amount of characters (two for example).
Unnecessary API calls should be avoided — if a user keeps typing, we don’t want to trigger a request on every keystroke.
Ongoing requests should be canceled when a new search is triggered.
A loading indicator should appear while waiting for a response.
Handling this complexity is very hard but becomes very easy with RxJS. But does that mean that we shouldn’t use Signals here at all? Not really, the best is to leverage the power of both.
Signals + RxJS: The Best of Both Worlds
Rather than seeing Signals and RxJS as competitors, think of them as complementary tools. Signals are great for simplifying state management, while RxJS remains essential for asynchronous event handling.
Let’s take the example above and put the combination of RxJS and Signals to the test. Let’s implement a GitHub user search with the help of Signals and RxJS:
@Component({
template: `
<input (input)="searchTerm.set($any($event.target).value)"/>
@if(!loading()){
<ul>
@for(user of users(); track user){
<li>{{ user }}</li>
}
</ul>
} @else {
<div>Loading...</div>
}
`
})
export class App {
#ghUsers = inject(GithubUserService);
searchTerm = signal<string>('');
loading = signal(false);
users = toSignal(
toObservable(this.searchTerm).pipe(
debounceTime(500),
distinctUntilChanged(),
filter((value) => value.length > 2),
tap(() => this.loading.set(true)),
switchMap((value) => this.#ghUsers.getGithubUsers(value)),
tap(() => this.loading.set(false))
), { initialValue: [] }
);
}
There’s a lot happening here — we have our template, we declare some Signals, and we inject the GithubUserService
. But the most interesting part is the users Signal. Let’s take a closer look.
users = toSignal(
toObservable(this.searchTerm).pipe(
debounceTime(500),
distinctUntilChanged(),
filter((value) => value.length > 2),
tap(() => this.loading.set(true)),
switchMap((value) => this.ghUsers.getGithubUsers(value)),
tap(() => this.loading.set(false))
), { initialValue: [] }
);
Here’s the magic: we start with a Signal but leverage RxJS and its operators for the async logic. Once we have the final processed data, we convert it back into a Signal for fine-grained change detection in the UI.
This approach keeps our code concise, readable, and performant — all while making the most of both RxJS and Signals.
Why not just use the
async
pipe instead of Signals? Well, Signals are future-proof and designed to seamlessly integrate with upcoming advancements in Angular’s change detection, including potential Signal-based components in future versions.
Signals or Streams? Making the Right Choice
There’s a lot of confusion in the Angular community about when to use Signals and when to use Streams (RxJS) or when to use both. Let’s clear that up.
I always use the following rules of thumb. I always start by picking Singals or RxJS with the help of the following question.

Before passing a value to the template, I always ensure it's a Signal. If it's already a Signal, great! If it's an Observable, I convert it using toSignal
instead of relying on the async
pipe.
Signals or RxJS?
Signals introduce exciting new possibilities and may replace RxJS in certain cases. However, RxJS remains invaluable for many use cases. Instead of choosing one over the other, leverage the best tool for the job—or even combine both when it makes sense.
Do you enjoy the theme of the code preview? Explore our brand new theme plugin
Skol - the ultimate IDE theme
Northern lights feeling straight to your IDE. A simple but powerful dark theme that looks great and relaxes your eyes.
Prepare yourself for the future of Angular and become an Angular Signals expert today!
Angular Signals Mastercalss eBook

Discover why Angular Signals are essential, explore their versatile API, and unlock the secrets of their inner workings.
Elevate your development skills and prepare yourself for the future of Angular. Get ahead today!
Get notified
about new blog posts
Sign up for Angular Experts Content Updates & News and you'll get notified whenever we release a new blog posts about Angular, Ngrx, RxJs or other interesting Frontend topics!
We will never share your email with anyone else and you can unsubscribe at any time!
Responses & comments
Do not hesitate to ask questions and share your own experience and perspective with the topic

Nivek
Google Developer Expert (GDE)
for Angular & Web Technologies
A trainer, consultant, and senior front-end engineer with a focus on the modern web, as well as a Google Developer Expert for Angular & Web technologies. He is deeply experienced in implementing, maintaining and improving applications and core libraries on behalf of big enterprises worldwide.
Kevin is forever expanding and sharing his knowledge. He maintains and contributes to multiple open-source projects and teaches modern web technologies on stage, in workshops, podcasts, videos and articles. He is a writer for various tech publications and was 2019’s most active Angular In-Depth publication writer.
58
Blog posts
2M+
Blog views
39
NPM packages
4M+
Downloaded packages
100+
Videos
15
Celebrated Champions League titles
You might also like
Check out following blog posts from Angular Experts to learn even more about related topics like Modern Angular !
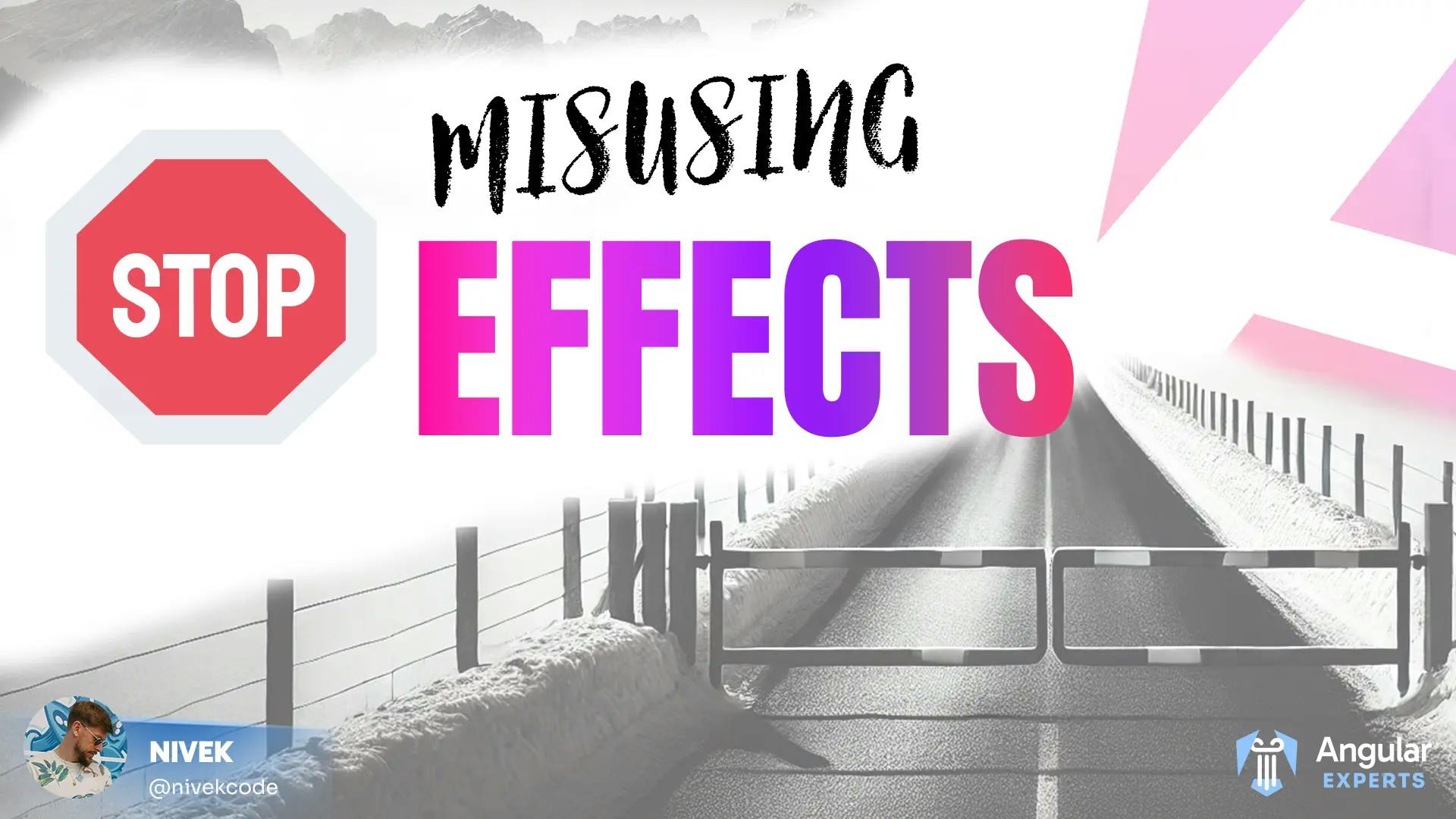
Stop Misusing Effects! Linked Signals Are the Better Alternative!
State synchronization with Angulars brand new linked Singlas API for a cleaner and more maintainable approach.
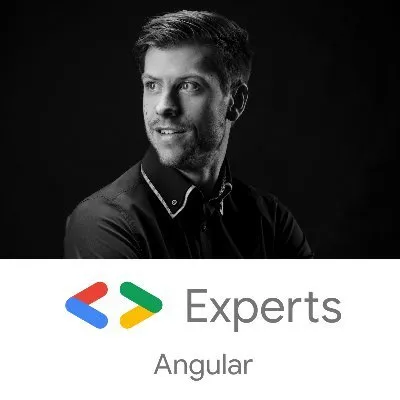
Kevin Kreuzer
@kreuzercode
Feb 10, 2025
4 min read

Hawkeye, the Ultimate esbuild Analyzer
Effortlessly analyze your JS bundles and uncover actionable insights to boost performance and enhance user experience.
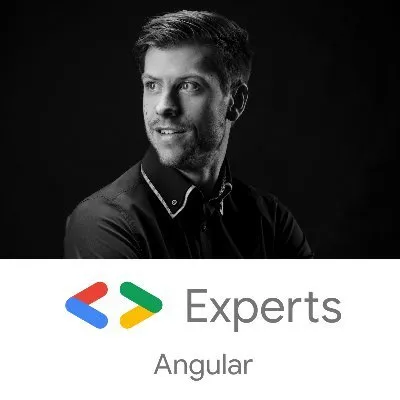
Kevin Kreuzer
@kreuzercode
Dec 28, 2024
10 min read
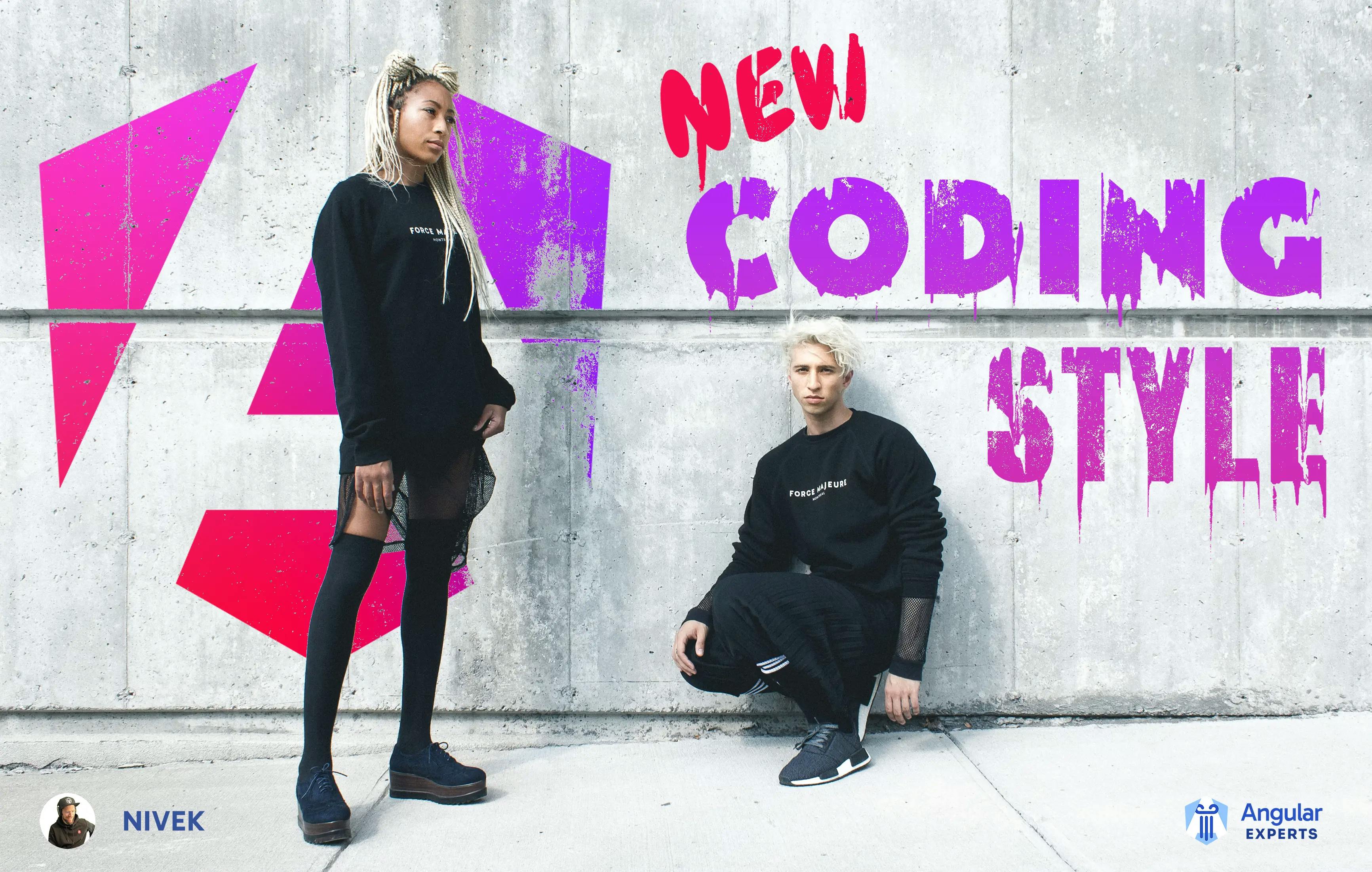
My new Angular Coding Style
Embracing the New Angular: A Fresh Perspective on how to write Angular code.
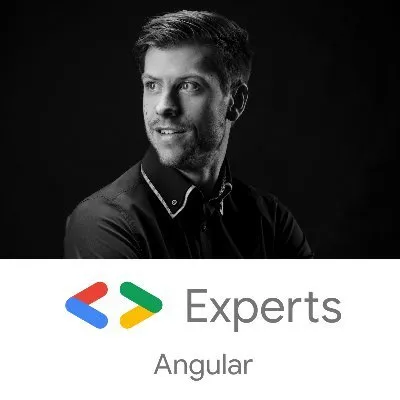
Kevin Kreuzer
@kreuzercode
Dec 2, 2024
7 min read
Empower your team with our extensive experience
Angular Experts have spent many years consulting with enterprises and startups alike, leading workshops and tutorials, and maintaining rich open source resources. We take great pride in our experience in modern front-end and would be thrilled to help your business boom