Improving DX with new Angular @Input Value Transform
Embrace the Future: Moving Beyond Getters and Setters! Learn how to leverage the power of custom transformers or the build in booleanAttribute and numberAttribute transformers.
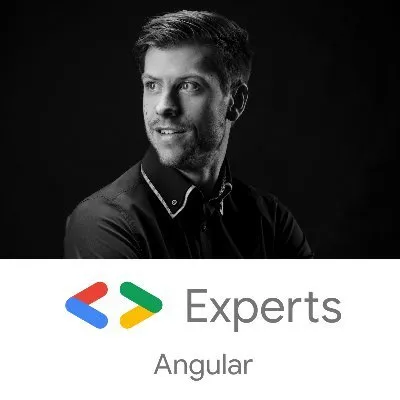
Kevin Kreuzer
@kreuzercode
Nov 18, 2023
3 min read

Angular 17 has rolled out, and it’s packed with some really cool stuff. There’s a bunch to get excited about, like the new control flow, deferred loading, and some slick improvements in server-side rendering.
But there’s this one feature that’s not making as much noise yet, and it’s pretty neat: @Input
Transforms. In this blog post, we’re going to take a look at this hidden gem and see how it can make handling input data in your apps a whole lot smoother. Let’s dive in and check out what this feature has to offer!
This feature has been available since version 16.1
@Input Transforms
Let’s take a look at a simple component that displays characters.
@Component({
standalone: true,
//...
template: `<h1>{{ character.name }}`
})
export class CharactersComponent {
@Input({ required: true }) character!: Character;
}
In our CharactersComponent
, we accept a required character @Input
. The goal is to display the character’s name in uppercase. With Angular 17, we can implement this feature by leveraging the new transform
feature.
Alternatively, we could also use a pipe for this transformation.
function characterNameUppercase(character: Character): Character {
return {
...character,
name: character.name.toUpperCase()
}
}
@Component({
standalone: true,
//...
template: `<h1>{{ character.name }}`
})
export class CharactersComponent {
@Input({ $required: true, transform: characterNameUppercase })
character!: Character;
}
In our example, we utilized a simple function that takes in a Character
and returns a transformed Character
with an uppercase name. The transform can be any function, which opens up a world of possibilities. Whether it’s formatting data, manipulating strings, or even more complex operations, everything is possible.
Build-in Transform Functions
Angular throws in two handy built-in transforms too — numberAttribute
and booleanAttribute
. Let’s take a quick look at how these built-in options can enhance our code.
numberAttribute
The numberAttribute
transformer is a transformer that converts any @Input
into a number
.
@Component({
standalone: true,
selector: 'circle',
//...
})
export class CircleComponent {
@Input({ transform: numberAttribute })
radius!: number;
}
With the numberAttribute
transform in place we can use the component in two manners.
<!-- does not compile without the numberAttribute transform -->
<circle radius="100"/>
<!-- works with or without the numberAttribute transform -->
<circle [radius]="100"/>
booleanAttribute
The other build-in directive is the booleanAttribute
function. A function that allows us to convert inputs to boolean
's.
This can be very useful since it optimizes how we can apply flags on components.
@Component({
standalone: true,
selector: 'product',
//...
})
export class ProductComponent {
@Input({ transform: booleanAttribute })
showDetails!: boolean;
}
With this code in place we can use the component in the following ways:
<!-- without the booleanAttribute this code would not compile -->
<my-product showDetails/>
<!-- without the booleanAttribute this code would not compile -->
<my-product showDetails="true"/>
<!-- works with and without the booleanAttribute transform -->
<my-product [showDetails]="true"/>
As you can see the booleanAttribute
function simplifies usage and makes it possible to simply enable a flag by adding the flag name.
If you like this feature you are not alone, Angular already utilizes this feature internally in the router.
Build-in Transform Downsides
While the built-in transforms offer nice functionality, it’s important to acknowledge some downsides. Utilizing these transforms leads to the loss of type definitions, making the code more susceptible to typographical errors. This increased vulnerability to typos can inadvertently introduce bugs into the system.
Let’s again take a look at the product.component
.
@Component({
standalone: true,
selector: 'my-product',
//...
})
export class ProductComponent {
@Input({ transform: booleanAttribute })
showDetails!: boolean;
}
We could use this component in the following way.
<my-product showDetails="falser"/>
In this scenario, not only is there a typographical error (we typed falser
instead of false
), but there’s likely also a bug. The goal seems to be to disable a flag, but due to the behavior of booleanAttribute
, which converts any string
not explicitly set to ”false”
into true
, the intended functionality may not be achieved as expected.
Integrating BooleanAttribute and NumberAttribute Into Existing Codebases
If you like this features you may want to use them accross your code base. But its a lot of effort, right? Well, don’t worry, I implemented some schematics to take care of this. The schematics will automatically add the booleanAttribute
and numberAttribute
transformers to inputs of type boolean
or number
. The schematics will merge the transformers with any existing input properties such as alias
or required
.
The schematics allow you to add either only
booleanAttribute
transformer or onlynumberAttribute
transformer or both.
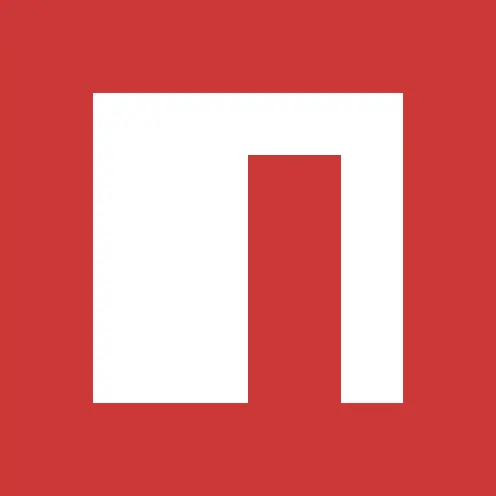
A transform transformer
Interested in the behind-the-scenes of this library? It was all put together live on my Twitch stream. Feel free to follow and join us for some relaxed, yet informative sessions on Angular and the latest in frontend development. Looking forward to seeing you there!
Do you enjoy the theme of the code preview? Explore our brand new theme plugin
Skol - the ultimate IDE theme
Northern lights feeling straight to your IDE. A simple but powerful dark theme that looks great and relaxes your eyes.
Prepare yourself for the future of Angular and become an Angular Signals expert today!
Angular Signals Mastercalss eBook

Discover why Angular Signals are essential, explore their versatile API, and unlock the secrets of their inner workings.
Elevate your development skills and prepare yourself for the future of Angular. Get ahead today!
Get notified
about new blog posts
Sign up for Angular Experts Content Updates & News and you'll get notified whenever we release a new blog posts about Angular, Ngrx, RxJs or other interesting Frontend topics!
We will never share your email with anyone else and you can unsubscribe at any time!
Responses & comments
Do not hesitate to ask questions and share your own experience and perspective with the topic

Nivek
Google Developer Expert (GDE)
for Angular & Web Technologies
A trainer, consultant, and senior front-end engineer with a focus on the modern web, as well as a Google Developer Expert for Angular & Web technologies. He is deeply experienced in implementing, maintaining and improving applications and core libraries on behalf of big enterprises worldwide.
Kevin is forever expanding and sharing his knowledge. He maintains and contributes to multiple open-source projects and teaches modern web technologies on stage, in workshops, podcasts, videos and articles. He is a writer for various tech publications and was 2019’s most active Angular In-Depth publication writer.
58
Blog posts
2M+
Blog views
39
NPM packages
4M+
Downloaded packages
100+
Videos
15
Celebrated Champions League titles
You might also like
Check out following blog posts from Angular Experts to learn even more about related topics like Angular !

Top 10 Angular Architecture Mistakes You Really Want To Avoid
In 2024, Angular keeps changing for better with ever increasing pace, but the big picture remains the same which makes architecture know-how timeless and well worth your time!
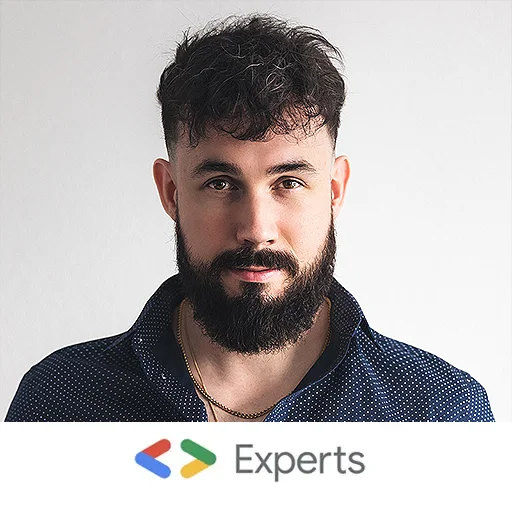
Tomas Trajan
@tomastrajan
Sep 10, 2024
15 min read

Angular Signal Inputs
Revolutionize Your Angular Components with the brand new Reactive Signal Inputs.
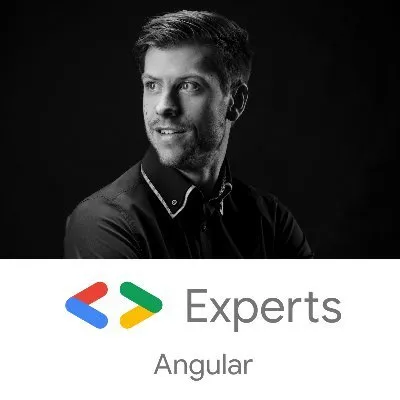
Kevin Kreuzer
@kreuzercode
Jan 24, 2024
6 min read
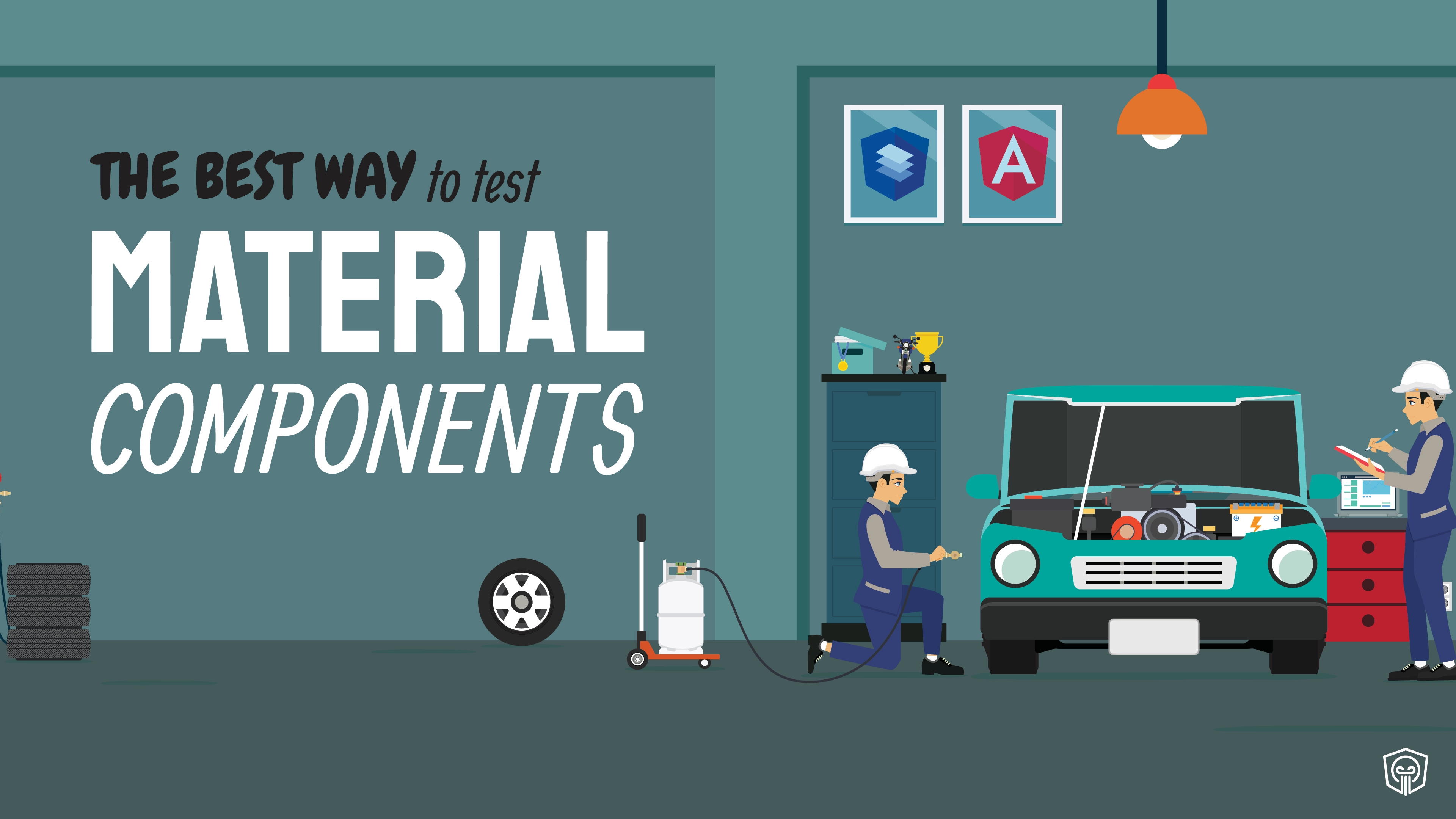
Angular Material components testing
How and why to use Angular Materials component harness to write reliable, stable and readable component tests
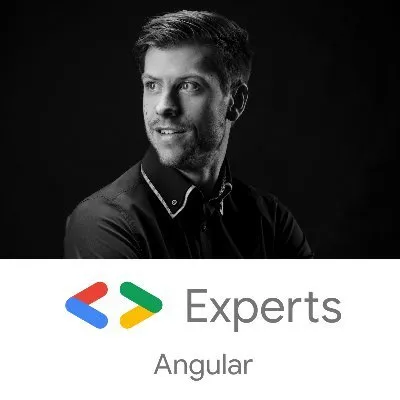
Kevin Kreuzer
@kreuzercode
Feb 14, 2023
7 min read
Empower your team with our extensive experience
Angular Experts have spent many years consulting with enterprises and startups alike, leading workshops and tutorials, and maintaining rich open source resources. We take great pride in our experience in modern front-end and would be thrilled to help your business boom