Angular Material components testing
How and why to use Angular Materials component harness to write reliable, stable and readable component tests
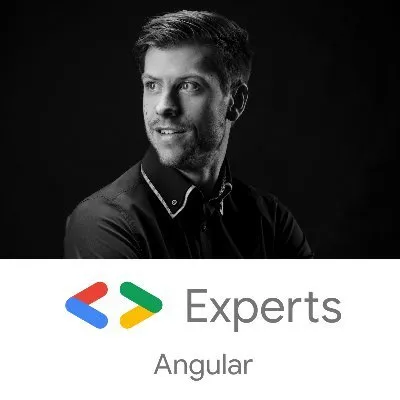
Kevin Kreuzer
@kreuzercode
Feb 14, 2023
7 min read
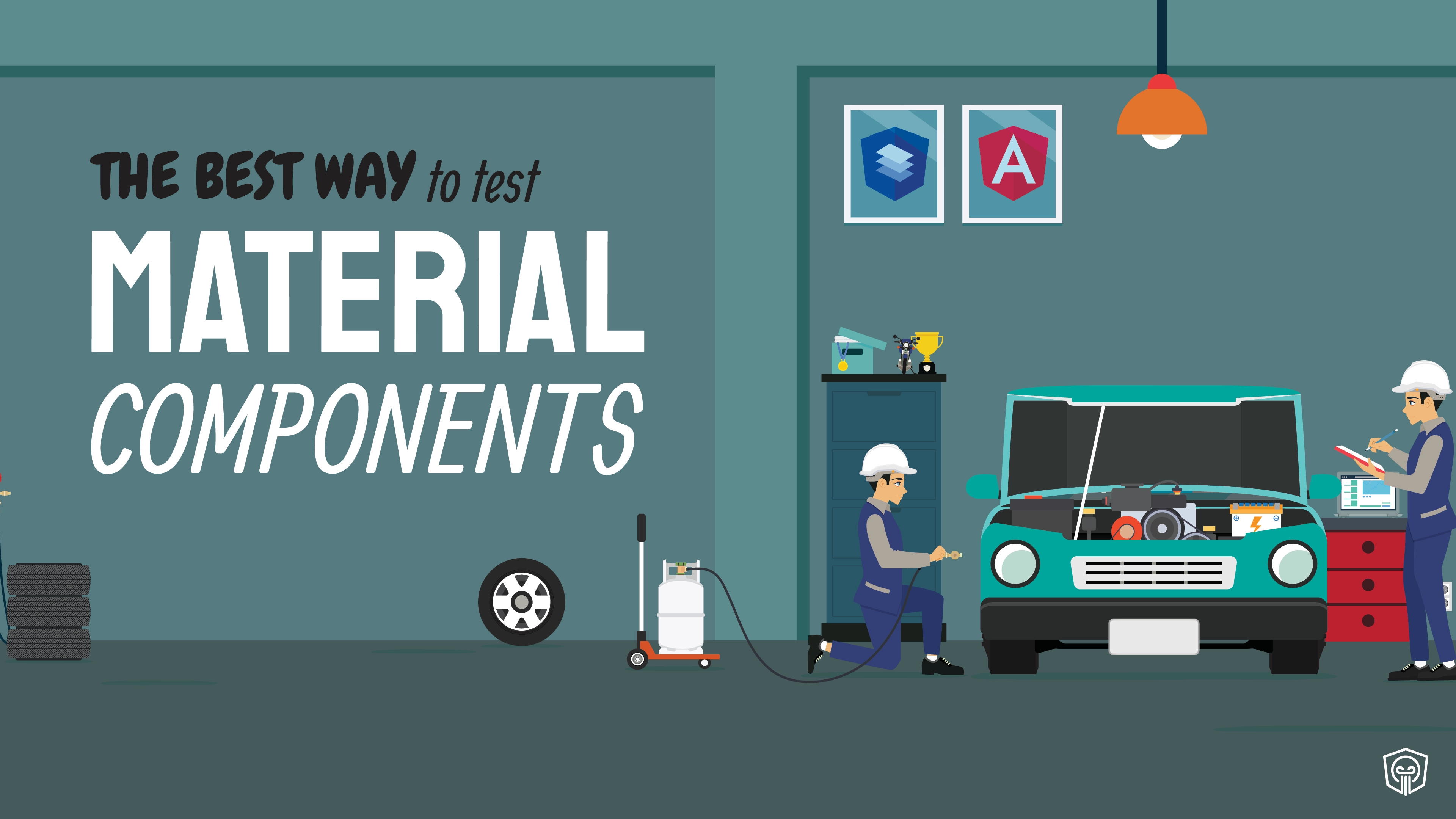
Good tests are the backbone of every application. They ensure that our app runs the way we expect it. Moreover, a robust test suite facilitates refactorings. Reliable tests allow us to change things under the hood without affecting the behavior.
In this article we want to show you the best way to test Angular material components.
Testing in Angular
In Angular, we distinguish between different types of tests; unit tests, component tests (integration tests) and end to end tests.
The testing pyramid for Angular applications
Unit tests are the most straightforward tests to write; they simply test functions of our TypeScript code. Component- and End to end tests on the other side are a little bit harder. They don’t only test the TypeScript code, but also the template, which may include third-party libraries such as Material.
What’s the problem with today's component tests? 🤔
When writing tests for components that include Angular material, we usually require a CSS query selector.
Let’s examine this further by having a closer look at some of the tests of a “Game of Thrones” filter demo application.

This application is pretty straight forward; it allows you to filter all characters by their “alive status” using the radio buttons. Additionally, we can also filter the characters by typing a search text in the input field.
For example, if we click on the radio button with the label “Dead”, the table is filtered accordingly.

Let’s write a unit test that tests that the radio button filter works. It tests that the correct method is called and the data source is filtered accordingly. We test the interaction between our template, Material and our Typescript logic.
it('should filter out the alive caracters if we set filter to dead', (done) => {
const deadRadio = fixture.debugElement.query(By.css('#deadFilter'));
const clickableElement = deadRadio.query(By.css('.mat-radio-container'));
clickableElement.nativeElement.click();
fixture.detectChanges();
fixture.whenStable().then(() => {
const rows = fixture.debugElement.queryAll(By.css('.mat-table tbody tr'));
expect(rows.length).toBe(5);
done();
});
});
test the filter feature by radio button on the GOT characters table
First, we get a hold of the radio button with the id deadFilter
. Unfortunately, nothing happens if we would execute a click on the radio button. Therefore, we query the radio button element to get the clickable element, which is the one with the mat-radio-container
class.
After we execute the click method on the clickable element we call fixture.detectChanges()
to indicate to the TestBed
to perform data binding. Next, we await the promise returned by fixture.whenStable()
. By awaiting the Promise, we are guaranteed that JavaScripts engine’s task queue has become empty.
At this point, we can get a hold of the table rows and assert its length. Since we are using a then handler we need to call Jasmine’s done callback to ensure that the test only finished after our assertions were executed.
There are a couple of downsides with this approach, maybe you already noticed them. 😉
We rely on internal implementation details
To check a radio button, it’s not enough to get a hold of it and call .click
on its nativeElement
. We need to find the clickable element inside the radio button itself.
const deadRadio = fixture.debugElement.query(By.css('#deadFilter'));
const clickableElement = deadRadio.query(By.css('.mat-radio-container'));
clickableElement.nativeElement.click();
test code to click on a material radio button
Querying material components by using CSS selectors is bad for multiple reasons;
First, we need to understand the material components. To find the clickable element, we need to dive into its internals.
Second, what if material adjusts the internal DOM structure of the radio button or just simply renames the mat-radio-container
class to mat-radio-box
. Our test would fail, even if our application is still running the way we expect it to, right?
Relying on implementation details of third party libraries is cumbersome because you are vulnerable to refactorings and you need to understand implementation details
Manually stabilize the fixture
A fixture becomes stable after Angular resolved all bindings and JavaScript’s task queue is empty. To guarantee a stabilized fixture, we need to remember to call fixture.detectChanges
and fixture.whenStable
.
fixture.detectChanges();
fixture.whenStable().then(() => {
// ...
});
Call fixture.detectChanges
and fixture.whenStable
to guarantee everything is rendered
Remember to call those statements is cumbersome. “If we forget it, we may end up in long and confusing debugging sessions” (burned child 😉).
Call done when you are done
As soon as we start to assert things in an asynchronous callback (for example with Promise or Subscriptions) we need to make sure to call the done function after our assertions. If we forget this, our assertion may not be executed. Means, our test may past, even if in reality, it doesn’t.
Tipp: When we work with promises we can also work with async/await statements instead of callbacks. Then we don’t have to call the done function.
Angular Material test harness to the rescue ⛑️
The “Harness concept” is inspired by the PageObject pattern. A harness class lets a test interact with a component over an official API.
By using component harnesses a test isolates itself against the internals of component library and resists internal refactorings.
Sounds good, how can we leverage materials test harness?
let loader: HarnessLoader;
beforeEach(() => {
fixture = TestBed.createComponent(FilterTableComponent);
component = fixture.componentInstance;
loader = TestbedHarnessEnvironment.loader(fixture);
fixture.detectChanges();
});
Setup a HarnessLoader
The first thing we need is a loader. Therefore we declare a variable in our top-level describe
and assign it inside the beforeEach
hook. We use the TestbedHarnessEnvironment
to get ourself a HarnessLoader
instance.
A
HarnessEnvironment
is the foundation of our harness test. There are different types of HarnessEnvironment’s. For Karma/Jasmine environment we use theTestbedHarnessEnvironment
.
That’s the full setup. Now, we can go on and take advantage of component harnesses in our test. Let’s rewrite the filter test we encountered previously.
it('should filter out the alive caracters if we set filter to dead', async () => {
const deadRadioButton = await loader.getHarness<MatRadioButtonHarness>(
MatRadioButtonHarness.with({ label: 'Dead' }),
);
const table = await loader.getHarness<MatTableHarness>(MatTableHarness);
await deadRadioButton.check();
const rows = await table.getRows();
expect(rows.length).toBe(5);
});
component test that tests the filter by radio button function with the help of Angular materials component harnesses
Look at how simple and readable our test has become! 🤩
When writing harness tests, we heavily work with async/await
. Therefore, one of the first things we should always do is to put the async keyword in front of our callback.
The loader allows us to load the harness objects. In our case, we are interested in the harness for the radio button with the “Dead” label. Once we got a hold of the harness, we can use its API to call the check method. The check method is self-explaining and internally knows how to check the checkbox — we no more need to lookup implementation details of mat-radio-button
.
The same goes for the table. We only have one table on our page, therefore it’s enough to ask the loader for the MatTableHarness
without specifying a selector. After we got a hold of the MatTableharness
we can use the getRows
function to get the number of rows.
Notice that we didn’t call fixture.detectChanges()
nor fixture.whenStable()
. The material component harness takes care of stabilizing the fixture once we interact with a component. This is amazing and makes our test less error-prone.
Another amazing benefit is the readability of our test. Take a look at the following two examples, what do you think? Which tests are easier to read? The classic tests or the harness test? Which one contain less boiler code?


Harness testing in Angular allows for easier, more readable and efficient component testing.
Do you enjoy the theme of the code preview? Explore our brand new theme plugin
Skol - the ultimate IDE theme
Northern lights feeling straight to your IDE. A simple but powerful dark theme that looks great and relaxes your eyes.
Do you enjoy the content and would like to learn more about how to ensure long term maintainability of your Angular application?
Angular Enterprise Architecture eBook

Learn how to architect a new or existing enterprise grade Angular application with a bulletproof tooling based automated architecture validation.
This will ensure that Your project stays maintainable, extendable and therefore with high delivery velocity over the whole project lifetime!
Get notified
about new blog posts
Sign up for Angular Experts Content Updates & News and you'll get notified whenever we release a new blog posts about Angular, Ngrx, RxJs or other interesting Frontend topics!
We will never share your email with anyone else and you can unsubscribe at any time!
Responses & comments
Do not hesitate to ask questions and share your own experience and perspective with the topic

Nivek
Google Developer Expert (GDE)
for Angular & Web Technologies
A trainer, consultant, and senior front-end engineer with a focus on the modern web, as well as a Google Developer Expert for Angular & Web technologies. He is deeply experienced in implementing, maintaining and improving applications and core libraries on behalf of big enterprises worldwide.
Kevin is forever expanding and sharing his knowledge. He maintains and contributes to multiple open-source projects and teaches modern web technologies on stage, in workshops, podcasts, videos and articles. He is a writer for various tech publications and was 2019’s most active Angular In-Depth publication writer.
58
Blog posts
2M+
Blog views
39
NPM packages
4M+
Downloaded packages
100+
Videos
15
Celebrated Champions League titles
You might also like
Check out following blog posts from Angular Experts to learn even more about related topics like Angular !

Top 10 Angular Architecture Mistakes You Really Want To Avoid
In 2024, Angular keeps changing for better with ever increasing pace, but the big picture remains the same which makes architecture know-how timeless and well worth your time!
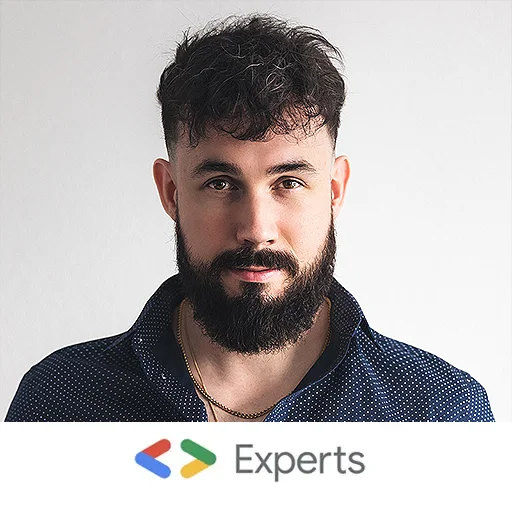
Tomas Trajan
@tomastrajan
Sep 10, 2024
15 min read

Angular Signal Inputs
Revolutionize Your Angular Components with the brand new Reactive Signal Inputs.
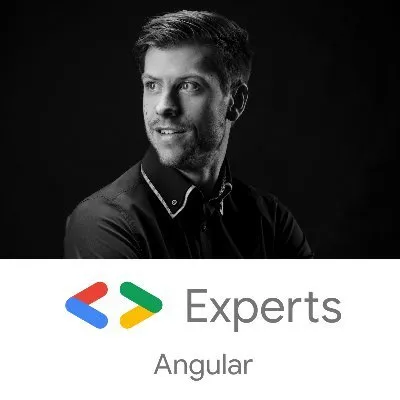
Kevin Kreuzer
@kreuzercode
Jan 24, 2024
6 min read

Improving DX with new Angular @Input Value Transform
Embrace the Future: Moving Beyond Getters and Setters! Learn how to leverage the power of custom transformers or the build in booleanAttribute and numberAttribute transformers.
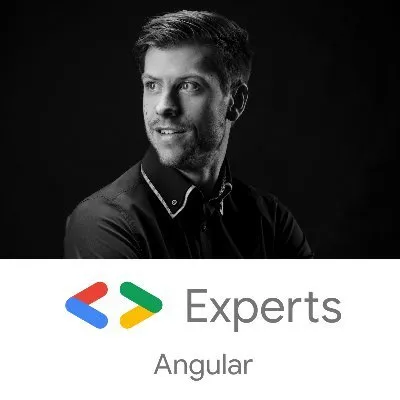
Kevin Kreuzer
@kreuzercode
Nov 18, 2023
3 min read
Empower your team with our extensive experience
Angular Experts have spent many years consulting with enterprises and startups alike, leading workshops and tutorials, and maintaining rich open source resources. We take great pride in our experience in modern front-end and would be thrilled to help your business boom